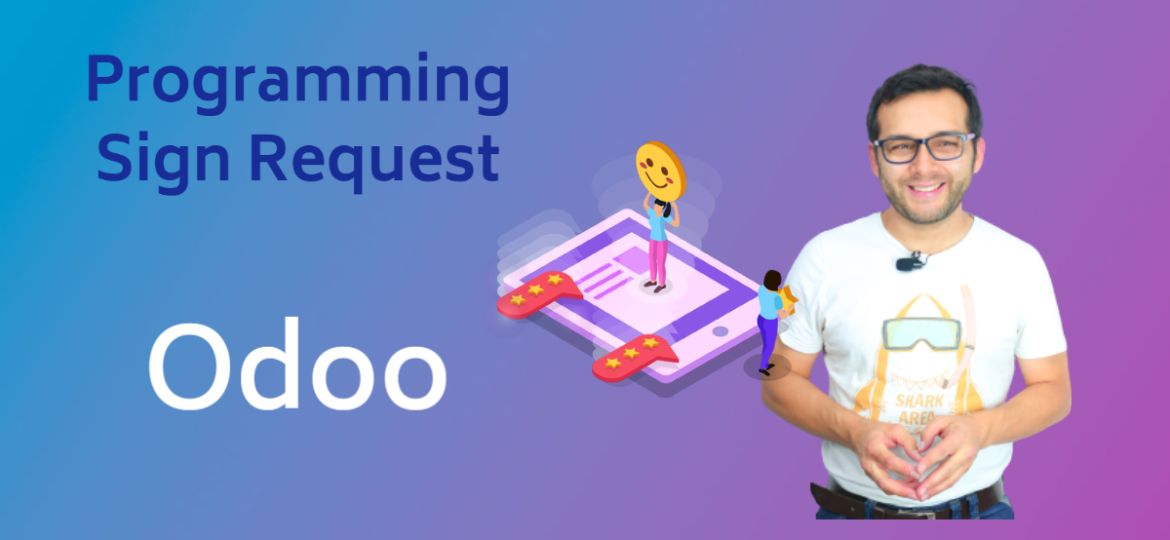
Odoo platform allows you to generate digital documents and send them via email as part of your business process, I.E. With the Inventory module, you can send to your Provider an email asking for a quotation for new products in a document to validate it, and then follow up the stock feed.
Another Document functionality with Odoo comes with the Sign module, with this module you can enable your PDF documents for signing employee contracts, and bills from customers and providers.
The Sign App works in situations you need to send the a sign request to a single person at time. In the case you need to send Sign Request massively you need to complement the App in a programmatically way.
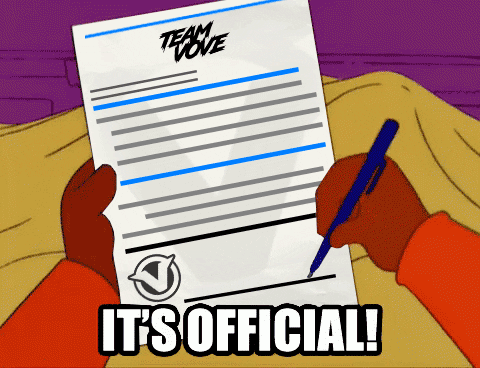
Maybe you are on a digital transformation project for your business or a for a customer, and is important to implements the the sign process and integrate with your IT solution, so, keep it reading and see how to use the Odoo API with the Sign module.
The code here is a Odoo API client writes in Python with the lib OdooRPC, you can find the documentation of the Lib here.
When I started, I found that there’s no developer documentation to Send sign request in Odoo, so, I spent several days checking Odoo server logs and the Inspect tool from web browser, finally I got the steps to send a Sign Request, gathering that, now is possible to implements in the Python client.
To send a Sign Request, Odoo follow 3 main steps to achieve that:
- Upload the PDF document from web browser (JS API client), get the ID of the document.
- Update the document with the fields to sign (Also API client).
- Prepare the email and send it with email data (subject, message) and recipients.
Upload the PDF document to Odoo Platform
Odoo get thet document in a JSON object with the document data and the PDF in Base64 format, so, assure to transform the PDF file to this format with base64 lib in Python.
#Code to connect to your Odoo instancen...n
pdf_to_attach = {
'name': 'document_name',
'type': 'binary',
'mimetype': 'application/pdf',
'store_fname': 'document_name',
'datas': pdf_base_64
}
# Upload document
attach_document = self.odoo.env['ir.attachment']
attachment_id = attach_document.create(pdf_to_attach)
‘attachment_id’, have the document id in Odoo, with this, you can send the Fields to Sign:
Update PDF document with Sign Field
In this JSON object you need to use the attachment_id value from the previous step.
Notice two things here:
- That attachment_id value is assigned to ‘template_id’ in JSON structure.
- The values ‘PosX’, ‘PosY’, ‘width’ and ‘height’ can vary to your desired position and size
sign_field = {
'type_id': 1,
'required': True,
'name': 'Sign',
'template_id': attachment_id,
'responsible_id': 3, # 1: Customer, 2: Company, 3: Employee, 4: Standard
'page': numpage,
'posX': 0.123,
'posY': 0.123,
'width': 0.123,
'height': 0.123
}
sign_item = self.odoo.env['sign.item']
item_id = sign_item.create(sign_field)
Prepare email and send the Sign request in Odoo
There is a variable called ’employee_id’ in ‘signer_ids’ field, get that value from Odoo with OdooRPC too.
email_fields = {
'template_id': template_id,
'signer_ids': [[0, 'virtual_25', {'role_id': 3, 'partner_id': employee_id}]],
'signer_id': False,
'signers_count': 1,
'has_default_template': True,
'follower_ids': [[6, False, []]],
'subject': 'Sign document request',
'filename': 'Filename',
'message_cc': 'email1@example.com', # Replace to your email
'attachment_ids': [[6, False, []]],
'message': '<p>This is a sign request, click the button<p>'
}
# Prepare email request
sign_email = self.odoo.env['sign.send.request']
email_id = sign_email.create(request_fields)
# Send email
request_sign = sign_email.send_request(email_id)
This code works, even though I’m confused with the other values [[0, ‘virtual_25’ , in signer_ids field, don’t know how Odoo works with this, I appreciate any information about that.
I published this for future reference for myself, also, you can use this code in the case you need to send several documents at once, just need to adapt to your needs.